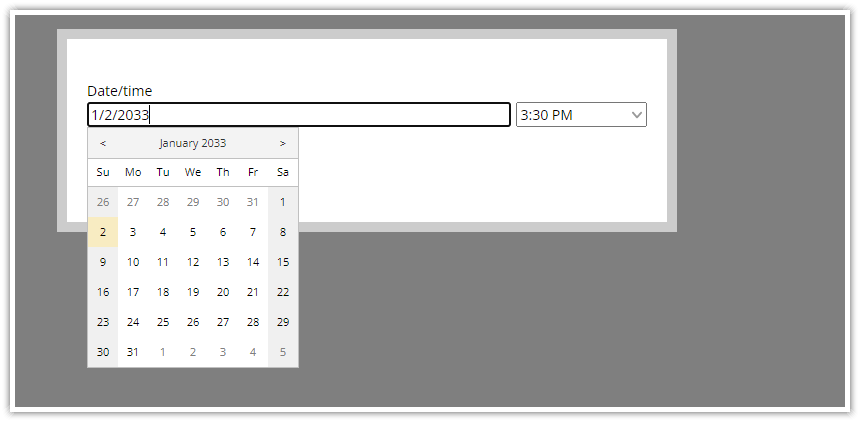
The datetime field (type: "datetime") displays a combined date/time field in the modal form() dialog.
Date/Time Field Properties
- type ("datetime") - specifies the form field type
- name (string) - the field name that will be displayed above the text box
- id (string) - the path that specifies the source/target property of the data object
- dateFormat (string) - specifies the date format using the syntax used by DayPilot.Date.toString(); optional
- timeFormat (string) - specifies the time format using the syntax used by DayPilot.Date.toString(); optional
- timeInterval (1 | 5 | 10 | 15 | 20 | 30 | 60) - specifies the time picker step size (in minutes); optional
- disabled (boolean) - makes the date field disabled (inaccessible and read-only); optional
- cssClass (string) - additional CSS class that will be applied to the form field; optional
- onValidate (function) - a callback function used to validate the field value; optional
Default Values
- If dateFormat is not specified, it uses the date format specified by the modal dialog locale.
- If timeFormat is not specified, it uses the time format specified by the modal dialog locale.
- If timeInterval is not specified, it uses 15 minutes.
Example
import {Modal} from "@daypilot/modal";
// ...
async function dateTime() {
const form = [
{
name: "Start",
id: "start",
dateFormat: "d.M.yyyy",
timeFormat: "H:mm",
type: "datetime",
},
];
const data = {
start: "2033-01-02T12:30:00",
};
const modal = await Modal.form(form, data);
console.log(modal.result);
}